The Read Resource Activity Class (Microsoft.ResourceManagement.Workflow.Activities.ReadResourceActivity) is used to read out a specific resource and its attributes from the FIM Portal database. Generally, this is used to pull out users, groups or other resources that you wish to use in a custom workflow. To read out a resource, all you need to know is the Resource ID and the attributes that you want to pull out.
In this example, I’m going to show you a very basic workflow that uses the Read Resource Activity to read a user from a request, then read attributes from that user’s manager. This will not only demonstrate how to read regular attributes, but also how we can read reference attributes from an object (and use them).
Step 1: Add a new Activity to your FIM Custom Activity Library
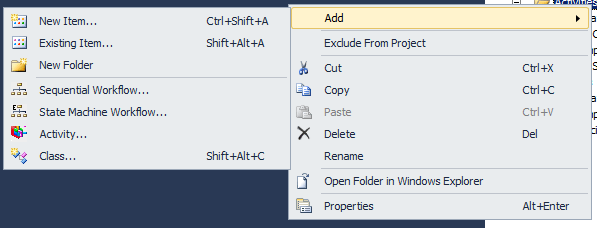
Step 2: Give that activity an appropriate name, in this case UpdateResourceExample.cs
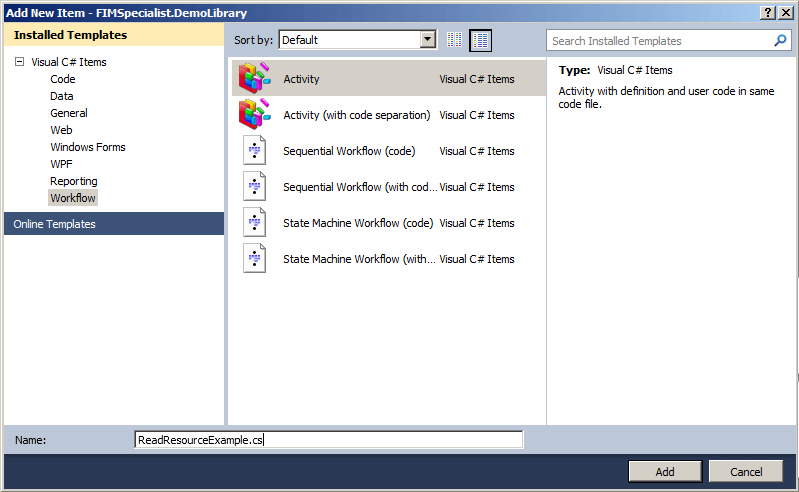
Step 3: Add the relevant activities into the workflow. In this example, we have added the Current Request Activity, two Read Resource activities and two code activities. The first read activity will read the user’s details, the second one will read the manager’s details. As this is simply a demonstration, we don’t really do anything with the manager in this workflow once we have them… but we could.
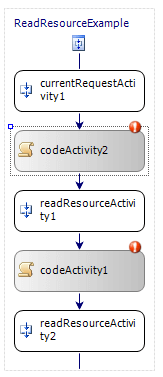
Step 4: Give the activities meaningful names. We’ll be using them in the code to configure these activities.
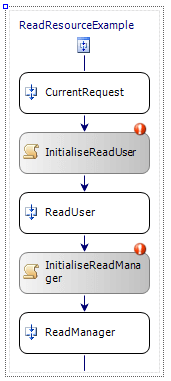
Step 5: Double click each of the code activities to promote the bindable properties for the code blocks.
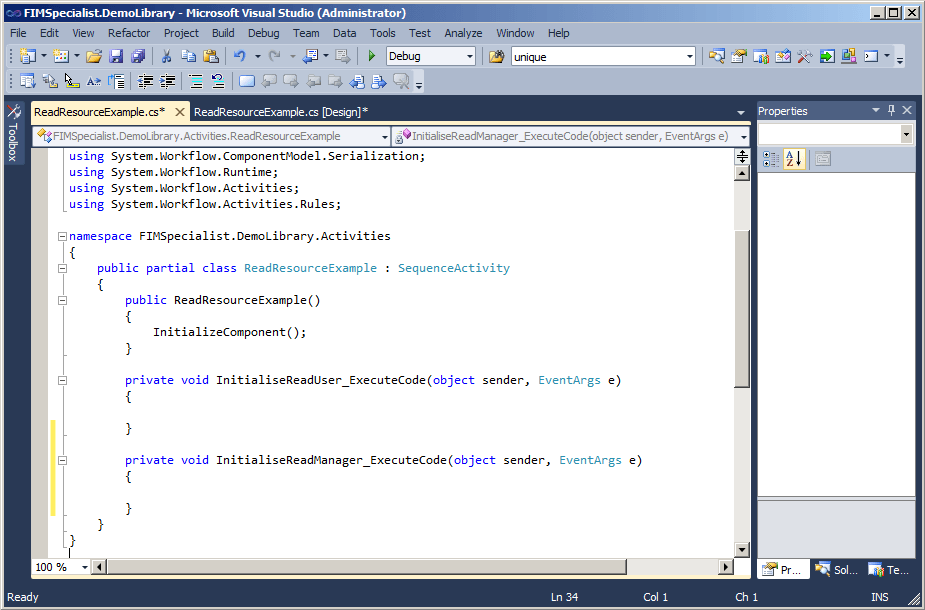
Step 6: Add the Microsoft.ResourceManagement references to your activity. In this example, we also add an include to Microsoft.ResourceManagement.WebServices so that we can use the UniqueIdentifier type for our Manager attribute

Step 7: Write the code to initialise the Read Resource Activity to pull out the FirstName, Lastname and Manager attributes for the current user. In this example, we are using the Current Request Activity to get the Resource ID of the user that we wish to query, however we could also omit this activity and get the current request’s target ID from the containing workflow (using SequentialWorkflow.TryGetContainingWorkflow(this, out containingWorkflow)).
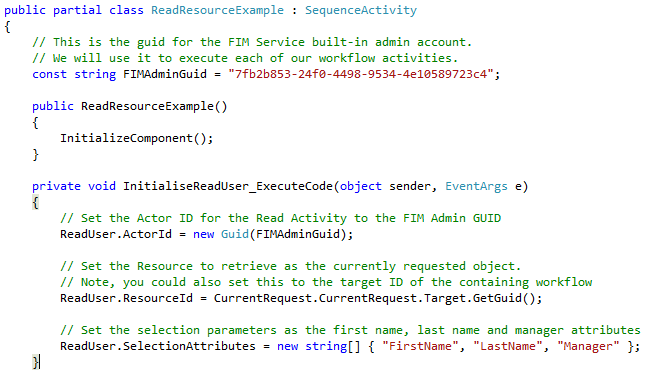
Step 8: In our sequential workflow, the next code activity occurs after the Read Resource Activity (which was initialised in the last step) has been executed. The Resource property on the ReadUser activity should now contain your retrieved user. We can put the data into usable variables, like so:
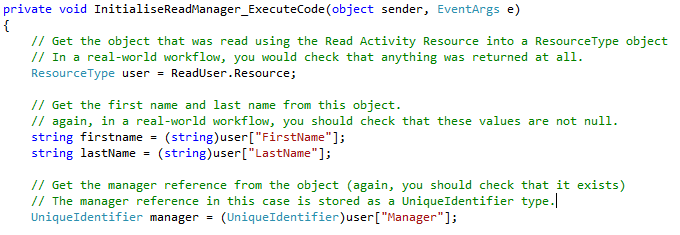
Step 9: The last step in our workflow is to initialise the ReadManager Activity (another Read Resource Activity). I’ve done this to basically show you how you can read out a current user’s reference attributes and use those attributes to perform an action on another object. For example, if your first Read Resource read out a group’s ExplicitMembers attribute, you could add a while loop activity into your workflow and perform an action on each member of the group.
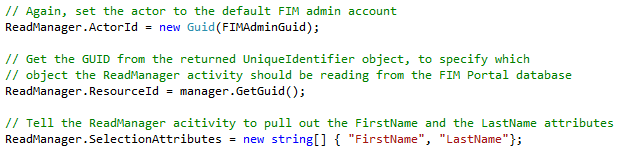
Step 10: Once the workflow is complete, we need to create a UI so that we can load it into the FIM Portal. Add a new class to your project.
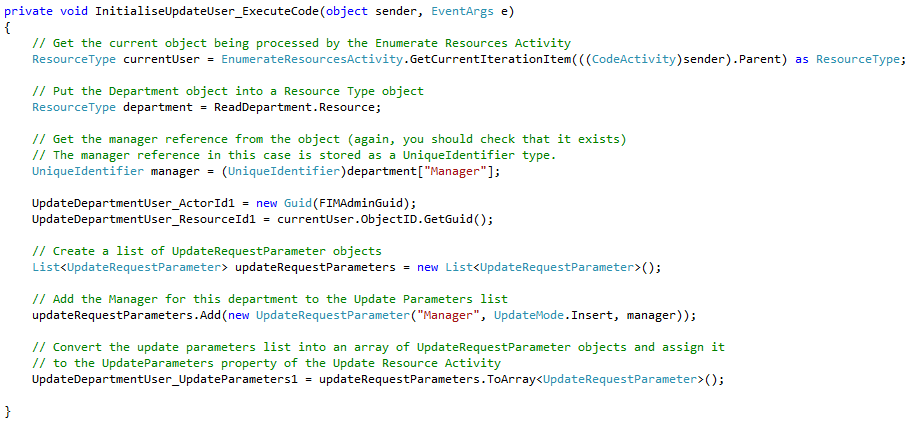
Step 11: Add the Microsoft.ReosurceManagement includes, an include to your activity (if it’s in a different namespace – if you’ve separated your project into Activity/WebUI folders as per the MS documentation, then it most likely will be) and then make the class implement the ActivitySettingsPart class.
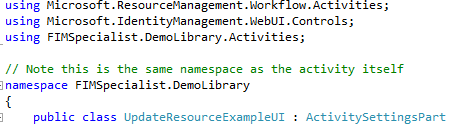
Step 12: Right click on ActivitySettingsPart and select “Implement Abstract Class” to generate the member methods which you must fill out.
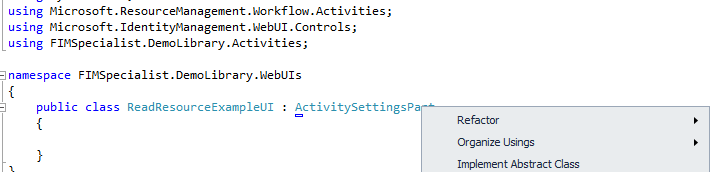
Once that’s been done, fill out the class as follows:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Microsoft.ResourceManagement.Workflow.Activities; using Microsoft.IdentityManagement.WebUI.Controls; using FIMSpecialist.DemoLibrary.Activities; namespace FIMSpecialist.DemoLibrary.WebUIs { public class ReadResourceExampleUI : ActivitySettingsPart { public override System.Workflow.ComponentModel.Activity GenerateActivityOnWorkflow(SequentialWorkflow workflow) { if (!this.ValidateInputs()) { return null; } ReadResourceExample readResource = new ReadResourceExample(); return readResource; } public override void LoadActivitySettings(System.Workflow.ComponentModel.Activity activity) { ReadResourceExample readResource = activity as ReadResourceExample; if (null != readResource) { } } public override ActivitySettingsPartData PersistSettings() { ActivitySettingsPartData data = new ActivitySettingsPartData(); return data; } public override void RestoreSettings(ActivitySettingsPartData data) { if (null != data) { } } public override void SwitchMode(ActivitySettingsPartMode mode) { bool readOnly = mode == ActivitySettingsPartMode.View; } public override string Title { // This is the title that we see when we are adding the activity to a workflow get { return "Read Resource Activity Example "; } } public override bool ValidateInputs() { return true; } } }
Only a very basic UI to load the Workflow is being used in this example, as we have no need to pull any additional workflow parameters into this workflow. In another tutorial, I will discuss how to create more advanced UI’s.
Step 13: Install the Workflow into the FIM Portal using GACUTIL (dev) or simply adding it to C:\windows\assembly using an administrator account (prod), and add the Activity Information Configuration item under Administration / All Resources in the FIM Portal. A future tutorial will detail how to do this. Your activity is now ready to be included in workflows.
Final code for the ReadResourceExample.cs file looks like this:
using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Collections; using System.Linq; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Compiler; using System.Workflow.ComponentModel.Serialization; using System.Workflow.Runtime; using System.Workflow.Activities; using System.Workflow.Activities.Rules; using Microsoft.ResourceManagement.WebServices.WSResourceManagement; using Microsoft.ResourceManagement.Workflow.Activities; using Microsoft.ResourceManagement.WebServices; namespace FIMSpecialist.DemoLibrary.Activities { public partial class ReadResourceExample : SequenceActivity { // This is the guid for the FIM Service built-in admin account. // We will use it to execute each of our workflow activities. const string FIMAdminGuid = "7fb2b853-24f0-4498-9534-4e10589723c4"; public ReadResourceExample() { InitializeComponent(); } private void InitialiseReadUser_ExecuteCode(object sender, EventArgs e) { // Set the Actor ID for the Read Activity to the FIM Admin GUID ReadUser.ActorId = new Guid(FIMAdminGuid); // Set the Resource to retrieve as the currently requested object. // Note, you could also set this to the target ID of the containing workflow ReadUser.ResourceId = CurrentRequest.CurrentRequest.Target.GetGuid(); // Set the selection parameters as the first name, last name and manager attributes ReadUser.SelectionAttributes = new string[] { "FirstName", "LastName", "Manager" }; } private void InitialiseReadManager_ExecuteCode(object sender, EventArgs e) { // Get the object that was read using the Read Activity Resource into a ResourceType object // In a real-world workflow, you would check that anything was returned at all. ResourceType user = ReadUser.Resource; // Get the first name and last name from this object. // again, in a real-world workflow, you should check that these values are not null. string firstname = (string)user["FirstName"]; string lastName = (string)user["LastName"]; // Get the manager reference from the object (again, you should check that it exists) // The manager reference in this case is stored as a UniqueIdentifier type. UniqueIdentifier manager = (UniqueIdentifier)user["Manager"]; // Again, set the actor to the default FIM admin account ReadManager.ActorId = new Guid(FIMAdminGuid); // Get the GUID from the returned UniqueIdentifier object, to specify which // object the ReadManager activity should be reading from the FIM Portal database ReadManager.ResourceId = manager.GetGuid(); // Tell the ReadManager acitivity to pull out the FirstName and the LastName attributes ReadManager.SelectionAttributes = new string[] { "FirstName", "LastName"}; } } }
Hello,
How can I run read resource activity for multiple resources ? WhileActivity and RepeatorActivity not working, the ReadActivity returns [resource = null]
thanks.
It has to do with the nesting. Once you nest the read resource activity inside another activity (eg, while), the object state isn’t maintained between activities. It’s weird.
I believe the only way I got around this was to promote the bindable properties of the Read Resource activity. Which just makes your code look horrible, but it seems to work.
Hi How can i retrieve data from workflow dictionary coming from previous workflow my data in InitilaiseReadManager_ExecuteCode function
Hi Ross,
First of all thank you for such easy going examples. I am very new into the custom workflow and activities stuff. While fetching user’s manager in the “InitializeReadManager_ExecuteCode”, i am getting error on “UniqueIdentifier”. It says, can’t resolved. Could you please tell me what possibly, I am doing wrong here??
Hi Ross,
I’m trying to use this example to develop a fairly simple workflow to address the issue that I raised on TechNet:
https://social.technet.microsoft.com/Forums/en-US/b9bf96b9-ac1f-4039-889d-8063fe45058f/mail-notification-with-blank-targetaccountname?forum=ilm2&prof=required
I wish to look of the presence of an attribute before sending a mail notification, else if the attribute is not present terminate the workflow, based on an IfElse activity.
I’m much more comfortable with VB.net, as opposed to C#. So I’m having some (noob) troubles!
The ReadUser and ReadManager objects that are described in your example, need to be defined within the code – I get errors stating that the name ReadUser does not exist in the current context. I get the fact that I need to create an object representing ReadUser, but am unsure which object type is valid – i.e. one that can contain those attributes that you describe in the example – ActorID, ResourceID and SelectionAttributes.
TIA,
Jon
Ah, OK.. I have that bit now.
I needed to add the following:
public Microsoft.ResourceManagement.Workflow.Activities.ReadResourceActivity ReadUser;
Jon
Ha, maybe not! When built I get further errors. I’ll stop messing for now, until I have some clear direction.
Ross,
Please ignore my ramblings, I was being a numpty! I think I’m on the right track with another TechNet article that you commented on.
Jon