The UocDropDownList control in the FIM Portal allows you to add drop-down lists to the create/edit RCDCs for your various object types. There are several ways in which you can populate these lists, and this page will attempt to describe these, from simplest to most complex:
1) Populate the options directly in the control
2) Use the attribute’s allowed values from the schema definition
3) Provide values via an XmlDataSource within the RCDC
4) Select resources from the Portal database to create a list of options
For the purposes of the following examples, we’re going to assume that you want to take the existing UocTextBox text box used to specify the Department attribute and convert it to a UocDropDownList.
<my:Control my:Name="Department" my:TypeName="UocTextBox" my:Caption="{Binding Source=schema, Path=Department.DisplayName}" my:Description=""> <my:Properties> <my:Property my:Name="Required" my:Value="{Binding Source=schema, Path=Department.Required}"/> <my:Property my:Name="Columns" my:Value="34"/> <my:Property my:Name="MaxLength" my:Value="128"/> <my:Property my:Name="Text" my:Value="{Binding Source=object, Path=Department, Mode=TwoWay}"/> </my:Properties> </my:Control>
1) Populate the options directly in the control
The most straight forward way to provide a set of options for a drop down list is to simply provide the options directly within the control. In this case, your control would look something like this:
<my:Control my:Name="Department" my:TypeName="UocDropDownList" my:Caption="{Binding Source=schema, Path=Department.DisplayName}" my:Description="{Binding Source=schema, Path=Department.Description}"> <my:Options> <my:Option my:Value="Accounting" my:Caption="Accounting" my:Hint="Accounting"/> <my:Option my:Value="Operations" my:Caption="Operations" my:Hint="Operations"/> <my:Option my:Value="Administration" my:Caption="Administration" my:Hint="Administration"/> </my:Options> <my:Properties> <my:Property my:Name="Required" my:Value="{Binding Source=schema, Path=Department.Required}"/> <my:Property my:Name="ValuePath" my:Value="Value"/> <my:Property my:Name="CaptionPath" my:Value="Caption"/> <my:Property my:Name="HintPath" my:Value="Hint"/> <my:Property my:Name="ItemSource" my:Value="Custom"/> <my:Property my:Name="SelectedValue" my:Value="{Binding Source=object, Path=Department, Mode=TwoWay}"/> </my:Properties> </my:Control>
2) Use the attribute’s allowed values from the schema definition
The next simplest way to populate a dropdown list is to access the localised allowed values defined by the attribute binding in the FIM Portal schema. You can see an example of how this is used by looking at the default RCDC for user creation, at the Employee Type attribute.
The first step in this method is to access the validation tab for the binding. In the FIM Portal, go to Administration->Schema Management->All Bindings. Then find the DepartmentUser binding. Select it and go to the validation tab. On this screen, type in your attribute’s possible values using the regex format: ^(Option 1|Option 2|Option 3|Option 4)?$.
In this example, we’ve created some very basic ones:
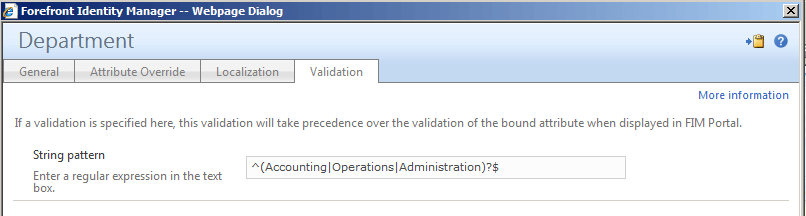
Now, to populate a drop down with these values, replace your existing Department control with the following:
<my:Control my:Name="Department" my:TypeName="UocDropDownList" my:Caption="{Binding Source=schema, Path=Department.DisplayName}" my:Description="{Binding Source=schema, Path=Department.Description}"> <my:Properties> <my:Property my:Name="Required" my:Value="{Binding Source=schema, Path=Department.Required}"/> <my:Property my:Name="ValuePath" my:Value="Value"/> <my:Property my:Name="CaptionPath" my:Value="Caption"/> <my:Property my:Name="HintPath" my:Value="Hint"/> <my:Property my:Name="ItemSource" my:Value="{Binding Source=schema, Path=Department.LocalizedAllowedValues}"/> <my:Property my:Name="SelectedValue" my:Value="{Binding Source=object, Path=Department, Mode=TwoWay}"/> </my:Properties> </my:Control>
3) Provide values via an XmlDataSource within the RCDC
You can also specify an XML Data Source at the start of your RCDC, which you can then link to a UocDropDownList using the itemSource Property. The ‘country’ attribute uses this capability in the default user creation RCDC.
First, update your RCDC to include an XMlDatasource at the top of the RCDC, after the ObjectDataSource references and before your first panel starts. In our departments example:
<my:XmlDataSource my:Name="departments"> <Departments> <Department Code="" Name=""/> <Department Code="ACC" Name="Accounting"/> <Department Code="ADM" Name="Administration"/> <Department Code="OPR" Name="Operations"/> </Departments> </my:XmlDataSource>
You then want to bind this to the itemSource of the Department control:
<my:Control my:Name="Department" my:TypeName="UocDropDownList" my:Caption="{Binding Source=schema, Path=Department.DisplayName}" my:Description="{Binding Source=schema, Path=Department.Description}"> <my:Properties> <my:Property my:Name="Required" my:Value="{Binding Source=schema, Path=Department.Required}"/> <my:Property my:Name="ValuePath" my:Value="Value"/> <my:Property my:Name="CaptionPath" my:Value="Caption"/> <my:Property my:Name="HintPath" my:Value="Hint"/> <my:Property my:Name="ItemSource" my:Value="{Binding Source=departments, Path=/Departments/*}"/> <my:Property my:Name="SelectedValue" my:Value="{Binding Source=object, Path=Department, Mode=TwoWay}"/> </my:Properties> </my:Control>
On the surface, this doesn’t seem much different to populating the options directly in control (as per option 1). However, by using the XmlDataSource, you are able to do some slightly advanced techniques, such as populating the dropdownlist dynamically. For example, if we had different classes of users, we could provide a different set of ‘department’ attributes that could be applied, as in this example:
<my:XmlDataSource my:Name="departments"> <Departments> <Staff> <Department Code="" Name=""/> <Department Code="ACC" Name="Accounting"/> <Department Code="ADM" Name="Administration"/> <Department Code="OPR" Name="Operations"/> </Staff> <Student> <Department Code="" Name=""/> <Department Code="ART" Name="Arts"/> <Department Code="ENG" Name="Engineering"/> <Department Code="HUM" Name="Humanities"/> </Student> </Departments> </my:XmlDataSource>
You then want to bind this to the itemSource of the Department control like so:
<my:Property my:Name="ItemSource" my:Value="{Binding Source=departments, Path=/Departments/%Attribute_EmployeeType%/*}"/>
Note that in this case we are assuming that the employee type attribute is already set, so this sort of dropdownlist is only really suitable for an “edit” RCDC, though I may cover later methods in which you can use this for a “create” RCDC.
4) Select resources from the Portal database to create a list of options
The last method I’ll present for populating a UocDropDownList supposes that you might want to provide a dynamic set of options based on objects that already exist in the FIM Portal – eg, if you created a separate Department object type in the schema, and wanted all of the department objects to appear in a drop down for selection. In fact, using this method, you can create a list of any single resource type in the FIM Portal – whether it’s a user, group, set, etc. The only downside is that there doesn’t seem to be a way to filter these results (that I’ve found).
The method was first hinted at in a suggestion made on the FIM Technet Forums by Henrik Nilsson and later expanded upon by Jacques Swanepoel. The only catch here is that even though each object’s display name will appear in the list, what you are actually populating the dropdownlist with are a series of object references, not string values – so you can’t store these directly into your string attribute (Department, in our example). What you can do, however, is store that reference in a separate attribute, and then use a simple workflow to dereference that object to get the display name (or any other attribute on the object) and assign the display name back into your string attribute.
Essentially, it works like this:
First, create the Department resource type in Administration->Schema Management and ensure that an MPR exists which gives the user access to read this resource.
Second, create a new reference attribute on your user object to store a reference back to one of those departments. In our example we will call it DepartmentReference.
Next, similar to the XmlDataSource, add a new ObjectDataSource to the top of your RCDC, after the TimeZoneDataSource. Very simply, this looks like this:
<my:ObjectDataSource my:TypeName="UocSearchDataSource" my:Name="search"/>
Lastly, edit your control to read all Department objects from that ObjectDataSource:
<my:Control my:Name="DepartmentReference" my:TypeName="UocDropDownList" my:Caption="{Binding Source=schema, Path=DepartmentReference.DisplayName}" my:Description="{Binding Source=schema, Path=DepartmentReference.Description}"> <my:Properties> <my:Property my:Name="Required" my:Value="{Binding Source=schema, Path=DepartmentReference.Required}"/> <my:Property my:Name="ValuePath" my:Value="Value"/> <my:Property my:Name="CaptionPath" my:Value="Caption"/> <my:Property my:Name="HintPath" my:Value="Hint"/> <my:Property my:Name="ItemSource" my:Value="{Binding Source=search, Path=Department}"/> <my:Property my:Name="SelectedValue" my:Value="{Binding Source=object, Path=DepartmentReference, Mode=TwoWay}"/> </my:Properties> </my:Control>
Note: We’re assigning a reference to a Department object (Binding Source=search,Path=Department) to the DepartmentReference field. Don’t get mixed up there.
Note 2: Without any ability to filter the results returned by the UocSearchDataSource, this is of limited use. If anybody has figured out how to do this, please let me know so that I can update this guide. For example, it’d be nice to be able to specify a “default group” that the user should go into, via a drop down, without having a list of every single group within the system. The UocIdentityPicker and UocListView aren’t always suitable (or elegant enough) for our purposes.
And that’s it, the four different ways to populate a UocDropDownList. If youhave any questions, please post a comment
Great article, thanks.
Although I had a problem with option
3) Provide values via an XmlDataSource within the RCDC
on FIM 2010 R2.
I have Divisions in my XmlDataSource:
To make my UocDropDownList control work, I had to change its properties as follows:
One more time with escaped html chars:
Great article, thanks.
Although I had a problem with option
3) Provide values via an XmlDataSource within the RCDC
on FIM 2010 R2.
I have Divisions in my XmlDataSource:
<my:XmlDataSource my:Name="divisions">
<Divisions>
<Division Code="1" Name="Name1"/>
<Division Code="2" Name="Name2"/>
</Divisions>
</my:XmlDataSource>
To make my UocDropDownList control work, I had to change according properties as follows:
<my:Property my:Name="ValuePath" my:Value="@Code"/>
<my:Property my:Name="CaptionPath" my:Value="@Name"/>
<my:Property my:Name="ItemSource" my:Value="{Binding Source=divisions, Path=/Divisions/*, Mode=OneWay}"/>
Thanks for this great article. It has made me have an easier time creating a drop down on FIM 2010. Though I have a question, how do I come up with an multiple identity picker that can aid users select more than one item… Else, can I have a drop-down with multi-selection ability in FIM 2010?
Thank you very much Ross for a great article!
Im trying, in any way I can to figure out how to do this on a create RCDC (Group creation). Do you have any advice?
How we can use this for a “create” RCDC.
How to do this on creation user page?